#include <iostream.h> int f1 (int, int, int); int f2 (int&, int&, int&); int f3 (int, int&, int); void main () { int i, j, k; i=1; j=2; k=3; cout << endl; cout << "Initial values of i, j, and k are: " << i << ", " << j << ", and " << k << endl << endl; cout << "f1(i,j,k) = " << f1(i,j,k) << endl; cout << "Values of i, j, and k after the call to f1 are: " << i << ", " << j << ", and " << k << endl << endl; cout << "f2(i,j,k) = " << f2(i,j,k) << endl; cout << "Values of i, j, and k after the call to f2 are: " << i << ", " << j << ", and " << k << endl << endl; cout << "f3(i,j,k) = " << f3(i,j,k) << endl; cout << "Values of i, j, and k after the call to f3 are: " << i << ", " << j << ", and " << k << endl; } int f1 (int x, int y, int z) { x=x+5; y=y+5; z=z+5; return(x+y+z); } int f2 (int& x, int& y, int& z) { x=x+5; y=y+5; z=z+5; return(x+y+z); } int f3 (int x, int& y, int z) { x=x+5; y=y+5; z=z+5; return(x+y+z); }
All Computer Programming languages and its basics (C,C++,JAVA,PHP,SAP(ALL MODULES)
Tuesday 15 April 2014
Call By Valuse and Call By reference
Calender
#include <iostream.h> #include <stdlib.h> #include <iomanip.h> typedef int Bool; // converts 0 to 'S', 1 to 'M', etc char whatDay (int); // not very good check for leap years Bool isLeapYear (int); // takes the number of the month, a flag saying whether year is leap int numOfDaysInMonth (int,Bool); void printHeader (int); // takes the number of the month, and the first day, prints, and updates // the first day of the next month void printMonth (int, int&); // prints the specified amount of spaces void skip (int); // prints leading spaces in monthly calendar void skipToDay (int); // terminates program in case of unrecoverable errors void disaster (); void main () { int year, firstDayInCurrentMonth; Bool leap; int currentMonth = 1; // start at Jan int numDays; cout << "What year do you want a calendar for? "; cin >> year; cout << "What day of the week does January 1 fall on?" << endl; cout << "(Enter 0 for Sunday, 1 for Monday, etc.) "; cin >> firstDayInCurrentMonth; leap = isLeapYear(year); skip(9); cout << year << endl; while (currentMonth <= 12) { numDays = numOfDaysInMonth(currentMonth,leap); printHeader(currentMonth); printMonth(numDays, firstDayInCurrentMonth); cout << endl << endl << endl; currentMonth = currentMonth + 1; } cout << endl; } void disaster () { cout << "Disaster! Exiting ..." << endl; exit (-1); } void skip (int i) { while (i > 0) { cout << " "; i = i - 1; } } char whatDay (int d) { if (d == 0) return('S'); else if (d == 1) return('M'); else if (d == 2) return('T'); else if (d == 3) return('W'); else if (d == 4) return('T'); else if (d == 5) return('F'); else if (d == 6) return('S'); else disaster(); } Bool isLeapYear (int y) { return ((y % 4) == 0); // simplified } void printHeader (int m) { if (m == 1) { skip(7); cout << "January" << endl; } else if (m == 2) { skip(7); cout << "February" << endl; } else if (m == 3) { skip(7); cout << "March" << endl; } else if (m == 4) { skip(7); cout << "April" << endl; } else if (m == 5) { skip(7); cout << "May" << endl; } else if (m == 6) { skip(7); cout << "June" << endl; } else if (m == 7) { skip(7); cout << "July" << endl; } else if (m == 8) { skip(7); cout << "August" << endl; } else if (m == 9) { skip(7); cout << "September" << endl; } else if (m == 10) { skip(7); cout << "October" << endl; } else if (m == 11) { skip(7); cout << "November" << endl; } else if (m == 12) { skip(7); cout << "December" << endl; } else disaster(); cout << " S M T W T F S" << endl; cout << "____________________" << endl; } int numOfDaysInMonth (int m, Bool leap) { if (m == 1) return(31); else if (m == 2) if (leap) return(29); else return(28); else if (m == 3) return(31); else if (m == 4) return(30); else if (m == 5) return(31); else if (m == 6) return(30); else if (m == 7) return(31); else if (m == 8) return(31); else if (m == 9) return(30); else if (m == 10) return(31); else if (m == 11) return(30); else if (m == 12) return(31); else disaster(); } void skipToDay (int d) { skip(3*d); } void printMonth (int numDays, int& weekDay) { int day = 1; skipToDay(weekDay); while (day <= numDays) { cout << setw(2) << day << " "; if (weekDay == 6) { cout << endl; weekDay = 0; } else weekDay = weekDay + 1; day = day + 1; } }
Grade
#include <iostream.h> #include <fstream.h> typedef int Bool; const Bool TRUE = 1; const Bool FALSE = 0; char printableBool (Bool i) { if (i==FALSE) return 'F'; else return 'T'; } int main () { ifstream f1, f2, f3check; ofstream f3; f1.open("exam"); if (!f1) { cout << "Cannot open file exam" << endl; return 1; } f2.open("key"); if (!f2) { cout << "Cannot open file key" << endl; return 1; } f3check.open("result"); if (f3check) { cout << "File result already exists" << endl; return 1; } f3.open("result"); if (!f3) { cout << "Cannot open file result" << endl; return 1; } int runningSum; int ans, key, score; runningSum = 0; f1 >> ans; if (!f1) { cout << "Error reading from file exam" << endl; return 1; } f2 >> key; if (!f1) { cout << "Error reading from file key" << endl; return 1; } if (ans == key) { runningSum = runningSum + 25; score = TRUE; } else score = FALSE; f3 << printableBool(score) << endl; if (!f3) { cout << "Error writing to file result" << endl; return 1; } f1 >> ans; if (!f1) { cout << "Error reading from file exam" << endl; return 1; } f2 >> key; if (!f1) { cout << "Error reading from file key" << endl; return 1; } if (ans == key) { runningSum = runningSum + 25; score = TRUE; } else score = FALSE; f3 << printableBool(score) << endl; if (!f3) { cout << "Error writing to file result" << endl; return 1; } f1 >> ans; if (!f1) { cout << "Error reading from file exam" << endl; return 1; } f2 >> key; if (!f1) { cout << "Error reading from file key" << endl; return 1; } if (ans == key) { runningSum = runningSum + 25; score = TRUE; } else score = FALSE; f3 << printableBool(score) << endl; if (!f3) { cout << "Error writing to file result" << endl; return 1; } f1 >> ans; if (!f1) { cout << "Error reading from file exam" << endl; return 1; } f2 >> key; if (!f1) { cout << "Error reading from file key" << endl; return 1; } if (ans == key) { runningSum = runningSum + 25; score = TRUE; } else score = FALSE; f3 << printableBool(score) << endl; if (!f3) { cout << "Error writing to file result" << endl; return 1; } f3 << endl << runningSum << endl; }
Merge Sort In C
#include <fstream.h> #include <iostream.h> int main () { ifstream f1, f2; ofstream f3; int i,j; f1.open("n1"); f2.open("n2"); f3.open("n1n2"); f1 >> i; f2 >> j; while (f1 && f2) { if (i < j) { while (i < j && f1 && f3) { f3 << i << endl; f1 >> i; } } else { while (j <= i && f2 && f3) { f3 << j << endl; f2 >> j; } } } while (f1) { f3 << i << endl; f1 >> i; } while (f2) { f3 << j << endl; f2 >> j; } return (0); }
Monday 14 April 2014
Average Of Two Numbers in C++
#include <fstream.h> void main () { ifstream f1; ofstream f2; f1.open("scores.96"); f2.open("final.96"); int s1, s2, s3; float w1, w2, w3; f1 >> s1 >> w1; f1 >> s2 >> w2; f1 >> s3 >> w3; f2 << (s1*w1+s2*w2+s3*w3); }
Decimal to binary conversion
Decimal to binary conversion
C program to convert decimal to binary: c language code to convert an integer from decimal number system(base-10) to binary number system(base-2). Size of integer is assumed to be 32 bits. We use bitwise operators to perform the desired task. We right shift the original number by 31, 30, 29, ..., 1, 0 bits using a loop and bitwise AND the number obtained with 1(one), if the result is 1 then that bit is 1 otherwise it is 0(zero).
C programming code
#include <stdio.h>
int main()
{
int n, c, k;
printf("Enter an integer in decimal number system\n");
scanf("%d", &n);
printf("%d in binary number system is:\n", n);
for (c = 31; c >= 0; c--)
{
k = n >> c;
if (k & 1)
printf("1");
else
printf("0");
}
printf("\n");
return 0;
}
OutPut Of Program:
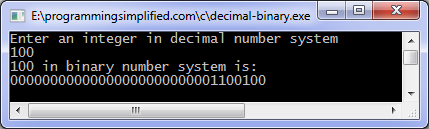
C program to convert decimal to binary: c language code to convert an integer from decimal number system(base-10) to binary number system(base-2). Size of integer is assumed to be 32 bits. We use bitwise operators to perform the desired task. We right shift the original number by 31, 30, 29, ..., 1, 0 bits using a loop and bitwise AND the number obtained with 1(one), if the result is 1 then that bit is 1 otherwise it is 0(zero).
C programming code
#include <stdio.h>
int main()
{
int n, c, k;
printf("Enter an integer in decimal number system\n");
scanf("%d", &n);
printf("%d in binary number system is:\n", n);
for (c = 31; c >= 0; c--)
{
k = n >> c;
if (k & 1)
printf("1");
else
printf("0");
}
printf("\n");
return 0;
}
OutPut Of Program:
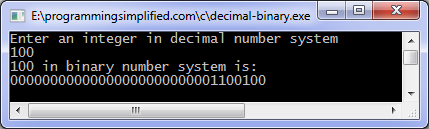
Sunday 13 April 2014
Pascle Triangle
public class Pascal
{
int i,j,k,l=1;
void PrintPascal(int n)
{
for (i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
System.out.print(" ");
}
for(k=0;k<i;k++)
{
System.out.print(i +" " );
}
System.out.println();
}
}
public static void main (String[] args)
{
Pascal p=new Pascal();
p.PrintPascal(5);
}
}
OUTPUT :
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
{
int i,j,k,l=1;
void PrintPascal(int n)
{
for (i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
System.out.print(" ");
}
for(k=0;k<i;k++)
{
System.out.print(i +" " );
}
System.out.println();
}
}
public static void main (String[] args)
{
Pascal p=new Pascal();
p.PrintPascal(5);
}
}
OUTPUT :
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
Batting and Bowling statistics
BATTING STATISTICS
- 733Runs
Michael Hussey
CSK
1Chris Gayle
RCB | 708 Runs
2Virat Kohli
RCB | 634 Runs
3 - 175*Runs
Chris Gayle
RCB
1David Miller
KXIP | 101* Runs
2Shane Watson
RR | 101 Runs
3 - 176.66Strike-Rate
Luke Wright
PWI
1David Miller
KXIP | 164.56
2AB de Villiers
RCB | 164.38
3
BOWLING STATISTICS
- 32Wickets
Dwayne Bravo
CSK
1James Faulkner
RR | 28 Wickets
2Harbhajan Singh
MI | 24 Wickets
3 - 5/16Wickets/Runs
James Faulkner
RR
1James Faulkner
RR | 5/20
2Jaidev Unadkat
RCB | 5/25
3 - 9.40Average
Zaheer Khan
RCB
1Chris Gayle
RCB | 13.33
2Sandeep Sharma
KXIP | 14.87
3
2013 STANDINGS
Team | Mat | Won | Lost | Tied | N/R | Net RR | For | Against | Pts |
CSK | 16 | 11 | 5 | 0 | 0 | 0.53 | 2461/303.5 | 2310/305.1 | 22 |
MI | 16 | 11 | 5 | 0 | 0 | 0.441 | 2514/318.1 | 2350/315.0 | 22 |
RR | 16 | 10 | 6 | 0 | 0 | 0.322 | 2405/310.5 | 2326/313.4 | 20 |
SH | 16 | 10 | 6 | 0 | 0 | 0.003 | 2166/308.5 | 2206/314.4 | 20 |
RCB | 16 | 9 | 7 | 0 | 0 | 0.457 | 2571/301.0 | 2451/303.1 | 18 |
KXIP | 16 | 8 | 8 | 0 | 0 | 0.226 | 2428/305.2 | 2417/312.5 | 16 |
KKR | 16 | 6 | 10 | 0 | 0 | -0.095 | 2290/313.4 | 2316/313.1 | 12 |
PWI | 16 | 4 | 12 | 0 | 0 | -1.006 | 2262/318.4 | 2519/310.5 | 8 |
DD | 16 | 3 | 13 | 0 | 0 | -0.848 | 2234/314.5 | 2436/306.4 | 6 |
Subscribe to:
Posts (Atom)
Comment
Comment Box is loading comments...